w15 <<
Previous Next >> C ex
w16
#include <gd.h>
#include <stdio.h>
#include <math.h>
#define WIDTH 800
#define HEIGHT 600
#define SCALE 100
//繪製電阻形狀
void drawResistor(gdImagePtr im, int x1, int y, int width, int height) {
int startX = x1 ;
int endX = x1 + width ;
// 第一段直線向前走一段
gdImageLine(im, startX, y, startX + width / 4 , y, gdImageColorAllocate(im, 0, 0, 255));
// 向上45度
gdImageLine(im, startX + width / 4 , y, startX + width / 4 + height / 2 , y - height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向下90度
gdImageLine(im, startX + width / 4 + height / 2, y - height / 2 , startX + width / 4 + height / 2 + height , y + height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向上90度
gdImageLine(im, startX + width / 4 + height / 2 + height, y + height / 2, startX + width / 4 + height / 2 + 2 * height, y - height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向下90度
gdImageLine(im, startX + width / 4 + height / 2 + 2 * height , y - height / 2, startX + width / 4 + height / 2 + 3 * height , y + height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向上90度
gdImageLine(im, startX + width / 4 + height / 2 + 3 * height, y + height / 2 , startX + width / 4 + height / 2 + 4 * height, y - height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向下90度
gdImageLine(im, startX + width / 4 + height / 2 + 4 * height, y - height / 2, startX + width / 4 + height / 2 + 5 * height, y + height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向上45度
gdImageLine(im, startX + width / 4 + height / 2 + 5 * height, y + height / 2, startX + width / 4 + height / 2 + 5 * height + height / 2, y, gdImageColorAllocate(im, 0, 0, 255));
gdImageLine(im, startX + width / 4 + height / 2 + 5 * height + height / 2, y, startX + width / 4 + height / 2 + 5 * height + height / 2 +5 , y, gdImageColorAllocate(im, 0, 0, 255));
{
int startX = 100;
gdImageColorAllocate(im, 0, 0, 255);
// 第一段直線向前走一段
gdImageLine(im, startX, y, startX + width / 4 , y, gdImageColorAllocate(im, 0, 0, 255));
// 向上45度
gdImageLine(im, startX + width / 4 , y, startX + width / 4 + height / 2 , y - height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向下90度
gdImageLine(im, startX + width / 4 + height / 2, y - height / 2 , startX + width / 4 + height / 2 + height , y + height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向上90度
gdImageLine(im, startX + width / 4 + height / 2 + height, y + height / 2, startX + width / 4 + height / 2 + 2 * height, y - height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向下90度
gdImageLine(im, startX + width / 4 + height / 2 + 2 * height , y - height / 2, startX + width / 4 + height / 2 + 3 * height , y + height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向上45度
gdImageLine(im, startX + width / 4 + height / 2 + 3 * height, y + height / 2, startX + width / 4 + height / 2 + 3 * height + height / 2, y, gdImageColorAllocate(im, 0, 0, 255));
gdImageLine(im, startX + width / 4 + height / 2 + 3 * height + height / 2, y, startX + width / 4 + height / 2 + 3 * height + height / 2 +5 , y, gdImageColorAllocate(im, 0, 0, 255));
}
{
int startX = 585;
gdImageColorAllocate(im, 0, 0, 255);
// 第一段直線向前走一段
gdImageLine(im, startX, y, startX + width / 4 , y, gdImageColorAllocate(im, 0, 0, 255));
// 向上45度
gdImageLine(im, startX + width / 4 , y, startX + width / 4 + height / 2 , y - height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向下90度
gdImageLine(im, startX + width / 4 + height / 2, y - height / 2 , startX + width / 4 + height / 2 + height , y + height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向上90度
gdImageLine(im, startX + width / 4 + height / 2 + height, y + height / 2, startX + width / 4 + height / 2 + 2 * height, y - height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向下90度
gdImageLine(im, startX + width / 4 + height / 2 + 2 * height , y - height / 2, startX + width / 4 + height / 2 + 3 * height , y + height / 2, gdImageColorAllocate(im, 0, 0, 255));
// 向上45度
gdImageLine(im, startX + width / 4 + height / 2 + 3 * height, y + height / 2, startX + width / 4 + height / 2 + 3 * height + height / 2, y, gdImageColorAllocate(im, 0, 0, 255));
gdImageLine(im, startX + width / 4 + height / 2 + 3 * height + height / 2, y, startX + width / 4 + height / 2 + 3 * height + height / 2 +5 , y, gdImageColorAllocate(im, 0, 0, 255));
gdImageLine(im, 100, y +20, 700, y +20, gdImageColorAllocate(im, 0, 255, 0));
}
}
int main() {
gdImagePtr im;
FILE *outputFile;
// 創建圖像物件
im = gdImageCreateTrueColor(WIDTH, HEIGHT);
if (im == NULL) {
fprintf(stderr, "Error creating GD image.\n");
return 1;
}
outputFile = fopen("1.png", "wb");
if (outputFile == NULL) {
fprintf(stderr, "Error opening the output file.\n");
return 1;
}
//配置顏色
int black, white, red, blue, green;
black = gdImageColorAllocate(im, 0, 0, 0);
white = gdImageColorAllocate(im, 255, 255, 255);
red = gdImageColorAllocate(im, 255, 0, 0);
blue = gdImageColorAllocate(im, 0, 0, 255);
green = gdImageColorAllocate(im, 0, 255, 0);
gdImageFilledRectangle(im, 0, 0, WIDTH - 1, HEIGHT - 1, white);
// 繪製牆面
gdImageLine(im, SCALE, SCALE, SCALE, HEIGHT - SCALE, black);
gdImageLine(im, WIDTH - SCALE, SCALE, WIDTH - SCALE, HEIGHT - SCALE, black);
// 繪製彈簧(電阻)
drawResistor(im, WIDTH / 3 + SCALE / 2, HEIGHT / 2, SCALE / 2, SCALE / 4);
// 繪製質量(方形)
gdImageFilledRectangle(im, WIDTH / 3 - SCALE / 2, HEIGHT / 2 - SCALE / 2, WIDTH / 3 + SCALE / 2, HEIGHT / 2 + SCALE / 2, red);
gdImageFilledRectangle(im, WIDTH * 2 / 3 - SCALE / 2, HEIGHT / 2 - SCALE / 2, WIDTH * 2 / 3 + SCALE / 2, HEIGHT / 2 + SCALE / 2, red);
gdImageFilledRectangle(im, 100, HEIGHT +300, 700, HEIGHT /2 +49 , black);
//生成圖片
gdImagePng(im, outputFile);
fclose(outputFile);
gdImageDestroy(im);
return 0;
}
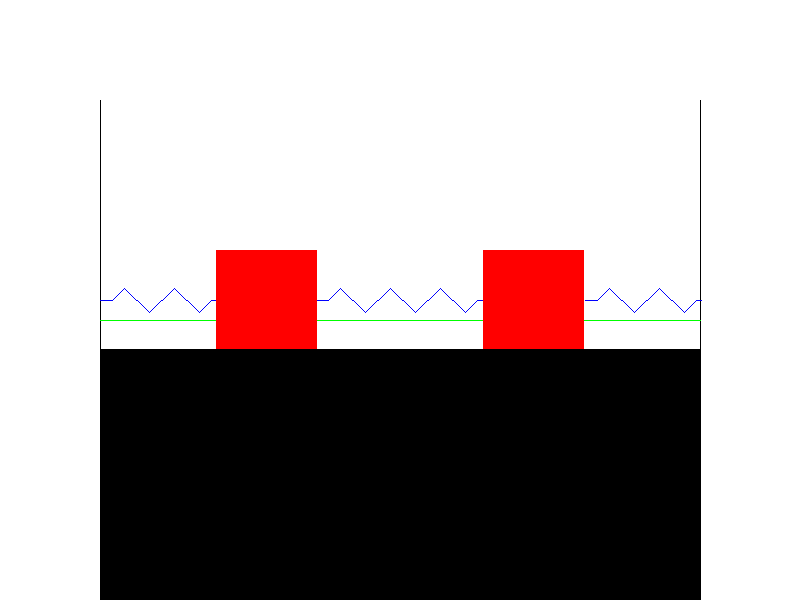
#include <stdio.h>
#include <math.h>
#define TIME_STEP 0.01 // 時間步長
#define SIMULATION_TIME 10.0 // 模擬時間
// 物體參數
#define m1 2.0
#define m2 3.0
#define k1 0.5
#define k2 1.0
#define k3 1.5
#define c1 0.25
#define c2 0.33
#define c3 0.5
#define X1_initial 1.0
#define X2_initial -0.5
#define V0 0.0
// 計算阻尼力
double dampingForce(double c, double v) {
return -c * v;
}
// 模擬物體運動
void simulateMotion() {
double X1 = X1_initial;
double X2 = X2_initial;
double V1 = V0;
double V2 = V0;
FILE *fp;
fp = fopen("motion_data.txt", "w");
for (double t = 0; t <= SIMULATION_TIME; t += TIME_STEP) {
double F1 = -k1 * (X1 - 0) - c1 * (V1 - 0); // 第一個物體受到的合力
double F2 = -k2 * (X2 - X1) - c2 * (V2 - V1); // 第二個物體受到的合力
double F3 = -k3 * (X2 - 0) - c3 * (V2 - 0); // 第二個物體受到的合力
double a1 = F1 / m1; // 第一個物體的加速度
double a2 = F2 / m2; // 第二個物體的加速度
// 更新速度和位置
V1 += a1 * TIME_STEP;
V2 += a2 * TIME_STEP;
X1 += V1 * TIME_STEP;
X2 += V2 * TIME_STEP;
// 在文件寫入時紀錄 m1 和 m2 的位置和速度數據
fprintf(fp, "%lf %lf %lf %lf %lf\n", t, X1, X2, V1, V2);
}
fclose(fp);
}
int main() {
simulateMotion();
return 0;
}
#include <stdio.h>
int main() {
FILE *gnuplotPipe = popen("gnuplot", "w");
if (gnuplotPipe) {
fprintf(gnuplotPipe, "set terminal png\n");
fprintf(gnuplotPipe, "set output 'motion_plot.png'\n");
fprintf(gnuplotPipe, "set xlabel 'Time'\n");
fprintf(gnuplotPipe, "set ylabel 'Position / Velocity'\n");
fprintf(gnuplotPipe, "plot 'motion_data.txt' using 1:2 with lines title 'm1 position', 'motion_data.txt' using 1:3 with lines title 'm2 position', 'motion_data.txt' using 1:4 with lines title 'm1 velocity', 'motion_data.txt' using 1:5 with lines title 'm2 velocity'\n");
fprintf(gnuplotPipe, "exit\n");
fflush(gnuplotPipe);
pclose(gnuplotPipe);
} else {
printf("Error opening pipe to Gnuplot.\n");
}
return 0;
}
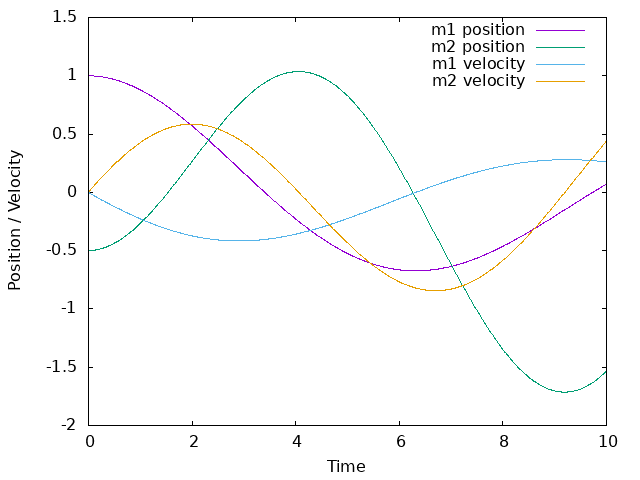
w15 <<
Previous Next >> C ex